I write prettier code than the formatter...
tl;dr
Autoformatting is great because it:
- Completely removes meaningless comments about formatting from Pull/Merge-requests.
- Allows you to free up mental resources which would otherwise be used on formatting.
- Allows you to write code faster.
Why readable code matters
Most software projects are long lived - most outlive their initial scope, and sometimes evolve into something entirely different. This is not due to errors in planning, but rather a consequence of business needs changing (which is a good thing. Imagine a company that never changes...)
The consequence of this is that code has to be reasonably easy to change and adapt to new purposes. It is therefore of paramount importance that code is easy to read. There is a famous quote by Robert C. Martin saying (roughly) that code is read 10 more than it is written.
Autoformatters work against readability
Almost every language has an autoformatter this day: rustfmt, prettier, csharpier, gofmt, etc. These programs partially take away control over code readability. Let us look at a common example:
// Manually formatted
let myList = [
{"name": "Joerge", "age": 43, "description": "I like long walks"},
{"name": "Catniss", "age": 23, "description": "I like to protect my people, and also sometimes to use the bow. I have developped excellent survival skills, and am practising using the knife for offensive purposes."},
{"name": "Gretchen", "age": 54, "description": "I have a hundred cats"}
];
// With autoformatter
let myList = [
{"name": "Joerge", "age": 43, "description": "I like long walks"},
{
"name": "Catniss",
"age": 23,
"description": "I like to protect my people, and also sometimes to use the bow. I have developped excellent survival skills, and am practising using the knife for offensive purposes."
},
{"name": "Gretchen", "age": 54, "description": "I have a hundred cats"}
];
Notice how the manually formatted example immediately makes it clear that we are dealing with a list of three items.
It seems like autoformatters make the code less readable!
Colleagues are worse than autoformatters
Few of us are writing code in isolation from the rest of the world. Enforcing consistent formatting across multiple persons is a nightmare: you will likely spend all your time writing style guides (that nobody reads), and doing reviews with meaningless formatting comments.
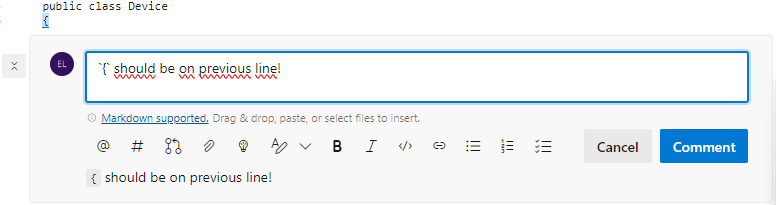
Let us be frank: Your colleagues suck at formatting code (and they probably think the same about you :)
Enter autoformatters. Nobody will be 100% pleased, but everybody will be consistent. I find that the decrease in readability from an autoformatter is a far lesser evil than the unstructured sphagetti caused by a colleague.
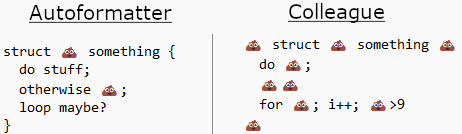
An autoformatter removes an entire class of comments from Merge/Pull-requests. This is especially important if you have junior developers on the team. There is a tendency for junior developers to only comment on "style" or "structure" when doing reviews. This is comfortable and easy to learn - but would it not be better to train them to try to understand customer requirements and problems in the code flow?
Freeing up mental space
After adopting formatters, something magical happens. You stop thinking about formatting when writing code. This frees up mental space to think about other things - like that infinite loop that you are just about to create.
Writing speed
Arguably, the speed with with one is writing code is less important than readability. However, improved writing speed is a nice side effect of formatters.
Style rules in linters
But why stop at autoformatters? For every review comment that could be automated, there is a linting rule waiting to be activated. Perhaps you would want your colleagues to...
- ...use sensible names for booleans
- ...name file after the exported class
- ...Never mix
async
and.then()
Like with autoformatters, you will sacrifice some control. But the gains are worth it ten times over.